Canvas Tkinter | Python 3
In this tutorial, we are going to see how to use Canvas in Tkinter. Canvas is a rectangular area for drawing images or other complex shapes. You can put graphics, text, widgets or frames on a Canvas.
Syntax:
Here is the syntax to create this widget:
c = Canvas ( master, option = value, ... )
Parameters:
- master : This represents the parent window.
- options : See below for a list of the most commonly used options for this widget. These options can be used as comma separated key-value pairs.
Example :
from tkinter import * master = Tk() w = Canvas(master, width=200, height=100) w.pack() w.create_line(0, 0, 200, 100, fill="red", dash=(4, 4)) w.create_line(0, 100, 200, 0) w.create_rectangle(55, 30, 140, 70, fill="red") mainloop()
Output:
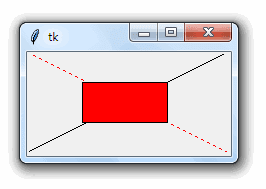
Canvas widget options
confine | If true (default), the Canvas cannot scroll outside the scroll region. |
cursor | Cursor used in the Canvas as an arrow, circle, point, etc. |
bd | Border width in pixels. The default value is 2. |
bg | Bbackground color. |
height | Canvas size in Y dimension. |
scrollregion | A tuple (w, n, e, s) that defines the scrolling area of the Canvas, where w is the left side, n is the top, e is the right side and s is the bottom. |
width | Canvas size in X dimension. |
highlightcolor | The color of the focus when the widget has the focus. |
relief | Relief indicates the type of border. Some of the values are SUNKEN, RAISED, GROOVE and RIDGE. |
The Canvas widget can support the following standard elements:
Arc: Create an arc.
coord = 15, 20, 200, 200 arc = w.create_arc(coord, start=0, extent=180, fill="red")
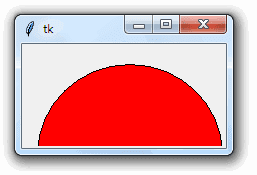
Image : Show an image on the Canvas.
from tkinter import * canvas = Canvas(width=300, height=200, bg='black') canvas.pack(expand=YES, fill=BOTH) # load the .gif image file gif1 = PhotoImage(file='python.gif') # put the image on the canvas canvas.create_image(50, 10, image=gif1, anchor=NW) mainloop()
Output:
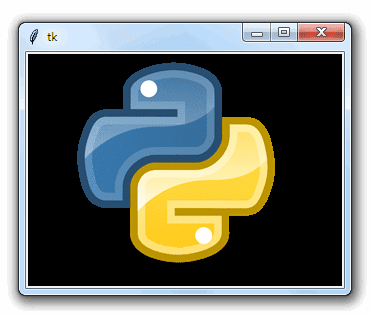
Line : Create a line.
line = canvas.create_line(x0, y0, x1, y1, options)
Oval : Creates a circle or ellipse with the given coordinates. Two pairs of coordinates are required; the upper left and lower right corners of the oval.
oval = canvas.create_oval(x0, y0, x1, y1, options)
Polygon : Create a polygon that must have at least three corners.
polygon = canvas.create_polygon(x0, y0, x1, y1, options)