Selection Sort in Python
We can create a Python program to sort the array elements using selection sort. In the selection sort algorithm, we look for the smallest element and put it in the right place. We swap the current element with the next smallest element.
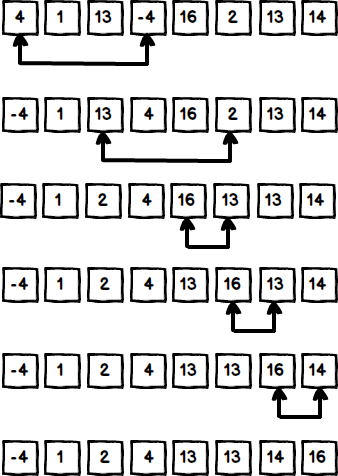
Selection Sort in Python
# Python script for implementing Selection Sort def selection_sort(arr): for i in range(len(arr)): # Find the min min = i for j in range(i+1, len(arr)): if arr[min] > arr[j]: min = j tmp = arr[i] arr[i] = arr[min] arr[min] = tmp return arr # Main program to test the above code arr = [98, 22, 15, 32, 2, 74, 63, 70] selection_sort(arr) print ("Sorted array: ") for i in range(len(arr)): print ("%d" %arr[i])
Output:
Sorted array: 2 15 22 32 63 70 74 98
Conclusion
The selection sort works best with a small number of elements. The worst-case execution complexity of the Selection sort is o(n2) similar to insertion and bubble sort.