How to Generate Random String in PHP
admin
0 Comments
generate random string in php without repetition, generate random string php, how to generate a random unique alphanumeric string in php, how to generate random alphanumeric string in php, how to generate random string in php, how to generate unique random string in php, php generate random alphanumeric string, php generate random number, php generate random string of characters, php random password generator, php random string unique
In this tutorial, we are going to see how to generate a random string in PHP. We can achieve that by storing all possible letters in a string and then generate a random index from 0 to the length of the string -1. As shown in the following code.
How to Generate Random String in PHP
<?php function getRandomStr($n) { // Store all possible letters in a string. $str = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $randomStr = ''; // Generate a random index from 0 to the length of the string -1. for ($i = 0; $i < $n; $i++) { $index = rand(0, strlen($str) - 1); $randomStr .= $str[$index]; } return $randomStr; } $n=25; echo getRandomStr($n); ?>
Output:
E1fMe0TwBJVvpzBeO0zL0jFJw
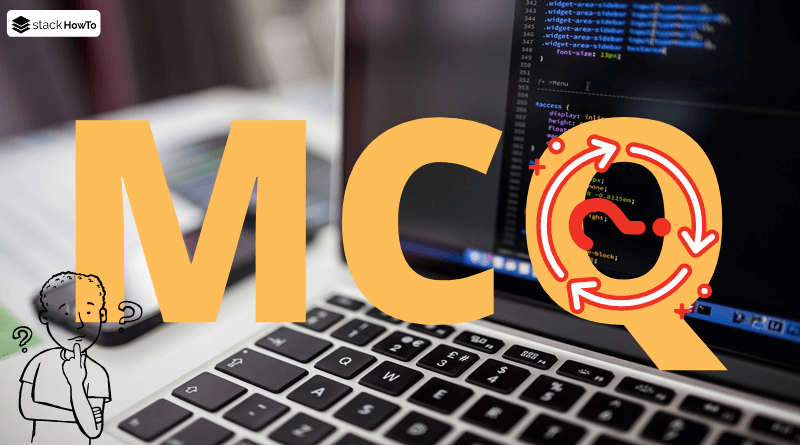
- Factorial Program in PHP Using Recursive Function
- Factorial in PHP Using For Loop
- How to Generate Random String in PHP
- Warning: Cannot modify header information – headers already sent
- How to send mail from localhost in PHP using WAMP server
- How to send mail from localhost in PHP using XAMPP
- How to Send an Email in PHP
- How to Validate an Email Address in PHP
- How to Check Format of Date in PHP
- How to check if a URL is valid in PHP
- How to Check Website Availability with PHP
- How to Generate Random Numbers in PHP
- How to Calculate Age from Date of Birth in PHP
- How to Extract Content Between HTML Tags in PHP
- How to check if file exists on remote server PHP
- How to get the client IP address in PHP
- How to Get IP Address of Server in PHP
- How to declare a global variable in PHP
- How to refresh a page with PHP
- How to get first day of week in PHP
- How to Change Max Size of File Upload in PHP
- How to compare two dates in PHP
- How to open a PDF file in browser with PHP
- How to Download file from URL using PHP
- How to remove an array element based on key in PHP
- How to get random value from an array in PHP
- How to calculate sum of an array in PHP
- How to remove null or empty values from an array in PHP
- How to Populate Dropdown List using Array in PHP
- How to get all the keys of an associative array in PHP
- How to get a value from an array in PHP
- How to remove a specific element from an array in PHP
- How to sort an associative array by value in PHP
- How to sort an associative array by key in PHP
- How to Check If a String Contains a Specific Word in PHP
- How to get the current date and time in PHP
- How to redirect to another page in PHP
- How to remove all spaces from a string in PHP
- Convert a date DD-MM-YYYY to YYYY-MM-DD in PHP
- Convert a date YYYY-MM-DD to DD-MM-YYYY in PHP
- How to get the current year in PHP
- How to convert a string to an integer in PHP
- How to get the first element of an array in PHP
- How to convert date to timestamp in PHP
- PHP: Compare two arrays and get the difference
- How to remove duplicates from an array in PHP
- How to sort an array in alphabetical order in PHP
- How to merge multiple arrays into one array in PHP
- How to add an element to the end of an array in PHP
- How to add an element to the beginning of an array in PHP
- How to remove the last element from an array in PHP
- How to remove the first element from an array in PHP
- How to replace part of a string in PHP
- How to count repeated words in a string in PHP
- PHP – Count Array Elements
- How to Reverse an Array in PHP
- How to check if a value exists in an array in PHP
- How to check if a key exists in an array in PHP
- How to reverse a string in PHP
- How to check if a variable is null in PHP
- How to check if a variable is empty in PHP
- How to check if a variable is undefined in PHP
- Convert HTML entities back to characters – PHP
- How to find the length of a string in PHP
- How to capitalize the first letter of a string in PHP
- PHP – Uppercase the first letter of each word in a string
- How to convert a string to lowercase in PHP
- How to compare two strings in PHP
- How to get the URL of the current page in PHP
- How to convert a string into an array in PHP
- How to extract a substring from a string in PHP
- How to count number of words in a string in PHP
- How to remove special characters from a string in PHP
- How to replace a word in a string in PHP
- How to Concatenate Two Strings in PHP
- How to count the number of characters in a string in PHP
- PHP – Remove spaces at the beginning and end of a string
- How to filter an array by value in PHP
- PHP – Get Domain Name from URL
- How to create a log file in PHP
- How to add days to a date in PHP
- PHP – Get file extension
- How to save an image from a URL in PHP
- How to Edit PHP ini File in Ubuntu Terminal
- How to increase the execution time of a PHP script
- How to enable cURL in PHP WAMP, XAMPP, and Ubuntu
- Convert Array to JSON in PHP
- PHP – Read JSON file
- How to display HTML tags as plain text using PHP
- PHP – Create JSON file
- How to convert result of MySQL query in JSON format
- PHP – Read CSV File into an Array
- How to convert an object to associative array in PHP
- How to extract or unzip a folder on FTP server in PHP
- How to Import CSV File in MySQL PHP
- Get Data From a Database Without Refreshing the Browser Using Ajax
- How to connect to Oracle database with PHP
- Connect to a PostgreSQL database with PHP PDO
- PHP – Convert Multidimensional Array to XML file
- What is PHP and how can I learn it?
- Hello World in PHP
- Comments in PHP
- How to Define a Variable in PHP
- PHP global variable with Example
- PHP Variable Scope
- PHP – Static Variables
- Type casting in PHP
- PHP Type Juggling
- Variable variables in PHP
- How to echo an array in PHP?
- How to Convert Decimal to Binary in PHP?
- How to Convert Binary to Decimal in PHP?
- How to assign multiple variables at once in PHP
- How to extract $_GET / $_POST parameters in PHP
- How to use $_GET in PHP?
- How to get a variable name as a string in PHP?
- How to print all defined variables and values in PHP
- PHP References: When to Use Them, and How They Work
- List of Superglobal Variables in PHP
- How to return a variable by name from a function in PHP
- How to delete a variable in PHP
- What is the difference between == and === in PHP
- How to check if a variable is NULL in PHP?
- How to check if an object is an instance of a specific class in PHP?
- How to check if a variable is a Boolean in PHP?
- How to check if a variable is a String in PHP?
- How to check if a variable is an Integer in PHP?
- How to check if a variable is a float in PHP?
- How to check if a variable is an array in PHP?
- How to check if a variable is undefined in PHP?
- How do you determine the data type of a variable in PHP?
- Difference between Include and Require in PHP
- How to combine HTML and PHP?
- How to add a new line within echo in PHP?
- How to display PHP variable values with echo, print_r, and var_dump
- The 6 best PHP frameworks in 2021
- PHP MCQ – Multiple Choice Questions and Answers – Basics – Part 1
- PHP MCQ – Multiple Choice Questions and Answers – Basics – Part 2
- PHP MCQ – Multiple Choice Questions and Answers – Basics – Part 3
- PHP Questions and Answers – Functions – Part 1
- PHP Questions and Answers – Functions – Part 2
- PHP Questions and Answers – Arrays – Part 1
- PHP Questions and Answers – Arrays – Part 2
- PHP Questions and Answers – Arrays – Part 3
- PHP Questions and Answers – Arrays – Part 4
- PHP Questions and Answers – Object-Oriented OOP – Part 1
- PHP Questions and Answers – Object-Oriented OOP – Part 2
- PHP Questions and Answers – Object-Oriented OOP – Part 3
- PHP Questions and Answers – String – Part 1
- PHP Questions and Answers – String – Part 2
- PHP Questions and Answers – Regular Expressions
- PHP Questions and Answers – Exception Handling