How to connect to Oracle database with PHP
In this tutorial, we are going to see how to connect to Oracle database with PHP. The majority of PHP applications have been developed with Oracle as the default database instead of MySQL. This is a very common problem for those using Oracle 11g in Windows (64-bit) with PHP applications that refuse the Oracle database connection. Here we will use Instant Client to connect Oracle database to our PHP application.
Instead of using a separate installation of PHP and Apache, we will use the WAMP server here. Just follow the steps to connect Oracle database to a PHP application on Windows platform (64 bits).
STEP 1: First install the WAMP server and Oracle 11g.
STEP 2: For Windows (64-bit), download instantclient-basic-windows.x64-11.2.0.4.0.zip, from this link and extract it to C:\Windows\SysWOW64\instantclient_11_2 and set it to the PATH environment variable
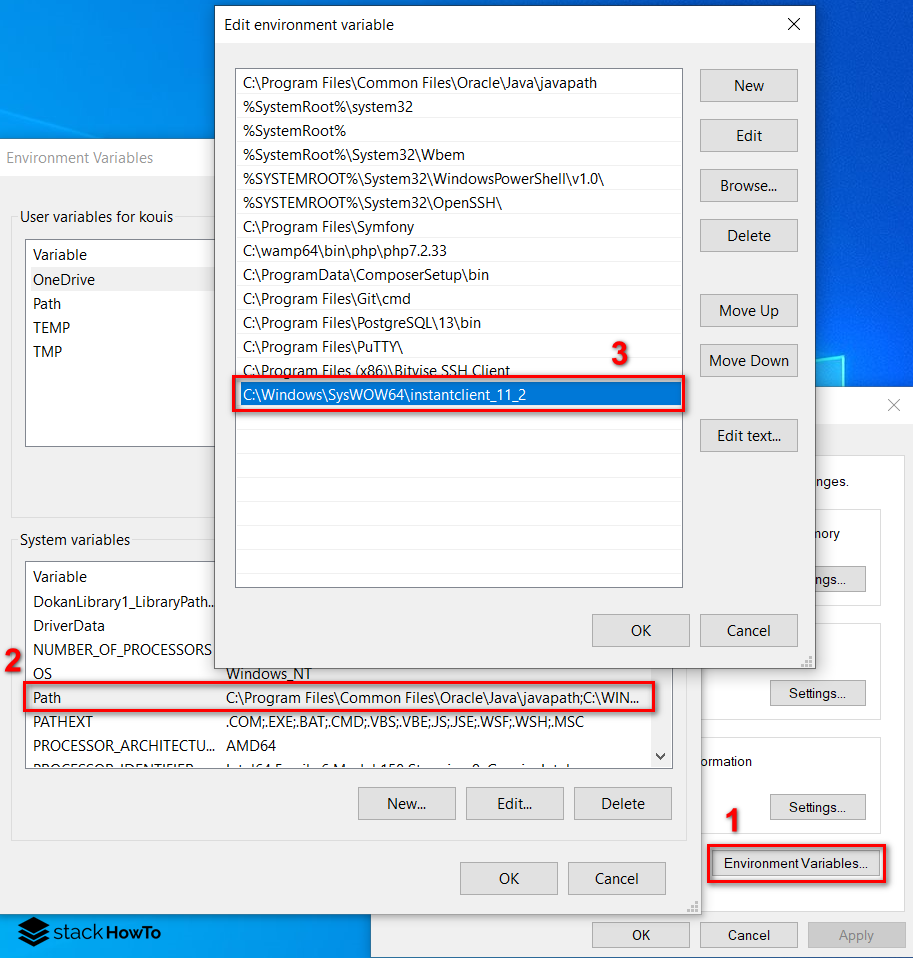
STEP 3: Restart the system.
STEP 4: To activate the PHP extension, edit the php.ini file and simply remove the semicolon “;” At the beginning of the line.
extension = php_oci8_11g.dll
Like the screenshot below:
STEP 5: Restart WAMP server.
STEP 6: Open the D:\Oracle11g\product\11.2.0\dbhome_1\network\admin\tnsnames.ora file to check the database name.
LISTENER_ORCL = (ADDRESS = (PROTOCOL = TCP)(HOST = localhost)(PORT = 1521)) ORACLR_CONNECTION_DATA = (DESCRIPTION = (ADDRESS_LIST = (ADDRESS = (PROTOCOL = IPC)(KEY = EXTPROC1521)) ) (CONNECT_DATA = (SID = CLRExtProc) (PRESENTATION = RO) ) ) ORCL = (DESCRIPTION = (ADDRESS = (PROTOCOL = TCP)(HOST = localhost)(PORT = 1521)) (CONNECT_DATA = (SERVER = DEDICATED) (SERVICE_NAME = orcl) ) )
Here the hostname is “localhost”, and the database name is “orcl”.
STEP 7: Now you can write your code to connect to Oracle database. Here is an example of PHP code:
<?php $conn = oci_connect('test_user', 'test_password', 'localhost/orcl'); if (!$conn) { die("Error when trying to connect to the database!"); } $stid = oci_parse($conn, 'SELECT * from users'); oci_execute($stid); echo "<table>"; echo "<tr><th>Name</th><th>Address</th></tr>"; while (($user = oci_fetch_array($stid, OCI_BOTH)) != false) { echo "<tr>"; echo "<td>".$user['NAME']."</td>"; echo "<td>".$user['Address']."</td>"; echo "</tr>"; } echo "</table>"; ?>
The hostname is taken from your tnsnames.ora file and the SID value is equal to your database name.