How to compare two dates in Javascript
admin
0 Comments
compare two dates, compare two dates in javascript, compare two dates in javascript yyyy mm dd, javascript compare date strings, javascript compare dates without time, javascript date greater than or equal to, js
In this tutorial, we are going to see how to compare two dates. In JavaScript we can compare two dates by converting them to a numeric value to match the times, for this we use the getTime() function. By converting the specified dates into numeric values, we can compare them directly.
We can use the relational operators below to compare two dates:
< > <= >=
We cannot apply these operators below on the Date object, but you can use it with the date.getTime() method:
== != !== ===
Example 1:
var date1 = new Date('2020-01-25'); var date2 = new Date('2020-01-20'); if(date1 > date2){ document.write('date1 is greater than date2'); } else document.write('date1 is less than date2');
Output:
date1 is greater than date2
Example 2:
var date1 = new Date('2020-01-20'); var date2 = new Date('2020-01-20'); if(date1.getTime() == date2.getTime()){ document.write('date1 and date2 are equal'); } else document.write('date1 and date2 are not equal');
Output:
date1 and date2 are equal
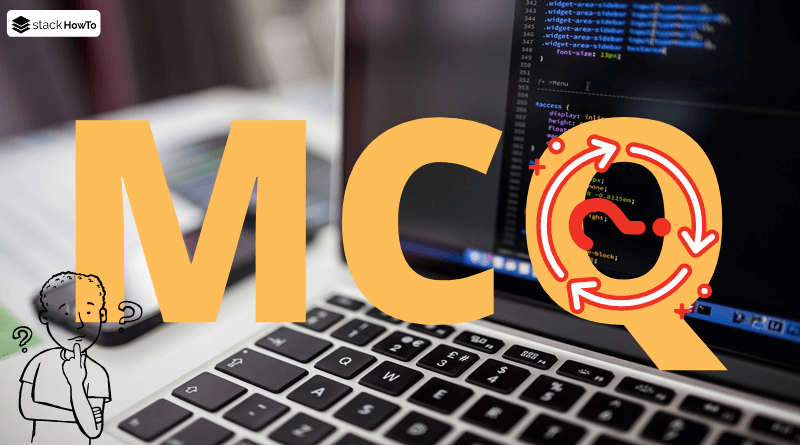
- What is a Web Worker in JavaScript?
- How to Sort an Array of Strings in JavaScript?
- ECMAScript vs JavaScript
- What is strict mode in JavaScript?
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 1
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 2
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 3
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 4
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 5
- JavaScript MCQs – Multiple Choice Questions and Answers – Part 6
- Arrow functions in JavaScript
- Difference between Node.js and AngularJS
- Difference between == and === in JavaScript
- What is JSON in Javascript
- How to go back to previous page in Javascript
- How to detect a mobile device with JavaScript
- How to close the current tab in a browser window using JavaScript
- How to convert input text to uppercase while typing using Javascript
- How to show a confirmation message before delete
- How to detect browser or tab closing in JavaScript
- How to get hash value from URL using JavaScript
- How to get the name, size, and type of a file in JavaScript
- Run javascript from the command line
- How to show an error message beside a field in HTML using Javascript
- Contact form with HTML, CSS, and Javascript
- Write your first Hello, World! in JavaScript
- How to get URL parameters in JavaScript
- Write a Javascript Program to Compute the Greatest Common Divisor GCD of Two Positive Integers
- How to calculate the square root of a number in JavaScript
- Convert decimal to binary, octal, or hexadecimal in Javascript
- Anagram in javascript
- How to Merge Objects in JavaScript
- Fibonacci Series Program in JavaScript
- How to refresh a page in Javascript
- Create an associative array in Javascript
- How to Format Number as Currency String in JavaScript
- How to disable right-click in JavaScript
- How to open a URL in a new tab in Javascript
- How to loop through a plain JavaScript object
- How to reverse a string in JavaScript
- Caesar Cipher in Javascript
- Generate a unique ID in Javascript
- SetInterval() and setTimeout() methods in Javascript
- Round to 2 decimal places in JavaScript
- Merge Sort in JavaScript
- How to remove a specific item from an array in Javascript
- Selection Sort in JavaScript
- Insertion Sort in JavaScript
- Bubble Sort in Javascript
- How to randomize (shuffle) an array in Javascript
- How to check if an object is an array in JavaScript
- Convert int to string in Javascript
- How to Get All Unique Values in a JavaScript Array
- How to copy an array in Javascript
- How to generate a random number in JavaScript
- Top JavaScript Frameworks to Use in 2022
- List of JavaScript functions
- Display a JavaScript variable in an HTML page
- Show a DIV after X seconds in Javascript
- Show/Hide multiple DIVs in Javascript
- How to change the text inside a DIV element in JavaScript
- How to get the text of HTML element in Javascript
- How to Append or Add text to a DIV using JavaScript
- Calculate age given the birth date in the format YYYYMMDD in Javascript
- How to compare two dates in Javascript
- Calculate the number of days between two dates in Javascript
- How to print a PDF file using Javascript
- Confirm password validation in JavaScript
- Check if an input field is a number in Javascript
- How to check if an input field is empty in Javascript
- How to validate an email address in JavaScript
- Remove the last character of a string in Javascript
- How to Get The Last Character Of a String in Javascript
- How to redirect to another page after 5 seconds in Javascript
- Digital Clock In JavaScript
- How to Display the Current Date and Time in Javascript
- Sum of two numbers in JavaScript
- How to find factorial of a number in JavaScript
- Function to check if a number is prime in Javascript
- How to reverse a number in JavaScript
- Code to check if age is not less than 18 years in Javascript
- How to Convert a String to Lowercase in Javascript
- How to Convert Strings to Uppercase in JavaScript
- Check if a number is a palindrome in JavaScript
- How to determine if a number is odd or even in JavaScript
- Armstrong Number in JavaScript
- Difference between PHP and Javascript
- How to pass JavaScript variables to PHP
- How to reset a form with JavaScript
- How to detect when a window is resized using JavaScript
- Call two functions from the same onClick event in JavaScript
- Adjust the iFrame height to fit with content in JavaScript
- How to get the value from the input field in Javascript
- How to add an element to the beginning of an array in JavaScript
- How to convert a JavaScript object to JSON string
- How to Generate a Timestamp in JavaScript
- How to Add Elements to an Array in JavaScript
- How to parse JSON into JavaScript
- How to Detect Screen Resolution with JavaScript
- How to Include a JavaScript File in another JavaScript File
- How to Get the Current URL with JavaScript
- How to return multiple values from a function in JavaScript
- How to sort an array of numbers in JavaScript
- How to check if a string is empty in JavaScript
- How to Convert Comma Separated String into an Array In JavaScript
- Difference between encodeURIComponent() and encodeURI() in JavaScript
- How To Decode a URL In JavaScript
- How To Encode a URL With JavaScript
- How to Create Multiline Strings in JavaScript
- How to change the background color with JavaScript
- How to check if a variable exists or is defined in JavaScript
- How to check if a variable is undefined or null in JavaScript
- How to trigger or pause a CSS animation in Javascript
- How to Increase and Decrease Image Size Using JavaScript
- How to change image size in JavaScript
- How to get image size (height & width) using JavaScript
- How to concatenate two string arrays in Javascript
- How to define a function in JavaScript?
- How to remove a specific element from an array in JavaScript
- How to remove duplicates from an array using Javascript
- How to check if a value exists in an array in JavaScript
- How to loop through an array in JavaScript
- How to split a string in JavaScript
- How to check if a string contains a substring in JavaScript
- How to replace all character in a string in JavaScript
- How to replace multiple spaces with single space in JavaScript
- How to replace all occurrences of a character in a string in JavaScript?
- What is JavaScript?
- 5 Best JavaScript Framework For 2021
- What Is AJAX?