Bubble Sort in Javascript
Sorting data in ascending or descending order can be done in different ways. In this tutorial, you will learn how to sort an array using the bubble sort algorithm.
In this method, smaller values gradually move upward, like an air bubble in water, and larger values move downward in the array.
In each loop, successive pairs of elements are compared and swapped if necessary. If the pair has the same value or is in ascending order, we keep it itself.
If there are N items to sort, the bubble sort does N-1 to cross the array.
Let’s look at an example, how it works.
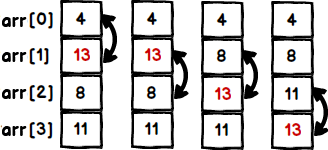
Script to sort array elements using bubble sort
function sort(arr){ var changed; do{ changed = false; for(var i=0; i < arr.length-1; i++) { if(arr[i] > arr[i+1]) { var tmp = arr[i]; arr[i] = arr[i+1]; arr[i+1] = tmp; changed = true; } } } while(changed); } var arr = [5, 8, 11, 6, 1, 9, 3]; sort(arr); console.log(arr);
Output:
[1, 3, 5, 6, 8, 9, 11]