Selection Sort in C
We can create a C program to sort the array elements using selection sort. In the Selection sort algorithm, we look for the smallest element and put it in the right place. We swap the current element with the next smallest element.
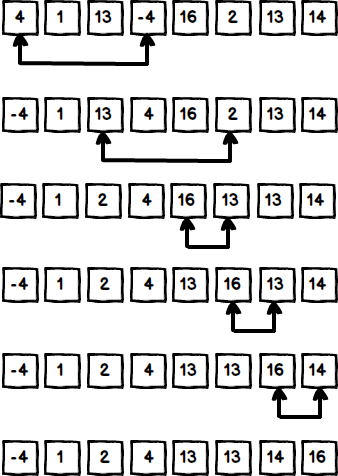
Selection Sort in C
/* Selection Sort in C */ #include <stdio.h> #define SIZE 10 int main() { int arr[10] = { 3, -2, 7, 10, -5, 22, 1, 27, 25, 30}; int i, j, tmp, index; //display the array elements for (i=0; i < SIZE; ++i) { printf("%4d", arr[i]); } for (i=0; i < (SIZE-1); i++) { index = i; for (j=i + 1; j < SIZE; j++) { if (arr[index] > arr[j]) index = j; } if (index != i) { tmp = arr[i]; arr[i] = arr[index]; arr[index] = tmp; } } printf("\n******** Array Sorted in Ascending Order ********\n"); for (i=0; i < SIZE; i++) printf("%4d", arr[i]); return 0; }
Output:
3 -2 7 10 -5 22 1 27 25 30 ******** Array Sorted in Ascending Order ******** -5 -2 1 3 7 10 22 25 27 30
Conclusion
The selection sort works best with a small number of elements. The worst-case execution complexity of the Selection sort is o(n2) similar to insertion and bubble sort.