Stack Implementation in C
In this tutorial, we are going to see how to implement a stack in C using an array. It involves various operations such as push, pop, etc.
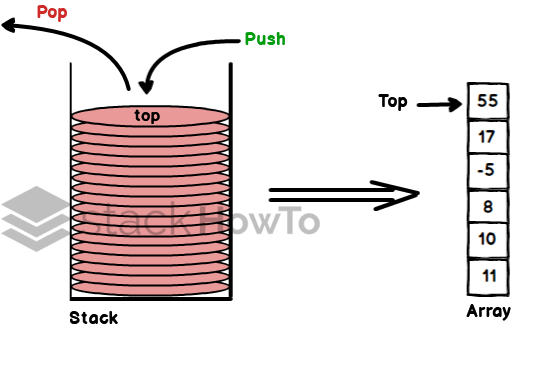
Stack Implementation in C
#include<stdio.h> #include<conio.h> #include<stdlib.h> #define size 5 struct stack { int arr[size]; int top; }; typedef struct stack STACK; STACK s; void push(int item) { if (s.top >= size - 1) return; s.top++; s.arr[s.top] = item; } int pop() { if (s.top == -1) return 1; int item; item = s.arr[s.top]; s.top--; return item; } void afficher() { int i; if (s.top == -1) printf("\nThe stack is empty!"); else { for (i = s.top; i >= 0; i--) printf("\n%d", s.arr[i]); } } int main() { int item, choice; int option = 1; s.top = -1; printf("\n\tStack implementation"); while (option) { printf("\nMain menu"); printf("\n1.Stack \n2.Unstack \n3.Print \n4.Exit"); printf("\nEnter your choice: "); scanf("%d", &choice); switch (choice) { case 1: printf("\nEnter The element to be stacked: "); scanf("%d", &item); push(item); break; case 2: item = pop(); printf("\nThe unstacked element is%d", item); break; case 3: afficher(); break; case 4: exit(0); } printf("\nDo you want to continue (Type 0(No) or 1(Yes))? : "); scanf("%d", &option); } return 0; }
Output:
Stack implementation Main menu 1.Stack 2.Unstack 3.Print 4.Exit Enter your choice: 1 Enter The element to be stacked: 50 Do you want to continue (Type 0(No) or 1(Yes))? : 1 Main menu 1.Stack 2.Unstack 3.Print 4.Exit Enter your choice: 3 50