Bubble Sort in C
Sorting data in ascending or descending order can be done in different ways. In this tutorial, we are going to see how to write a C program to sort an array using the bubble sort algorithm.
In this method, the smaller values gradually move up, like an air bubble in water, and the larger values move down the array.
In each loop, successive pairs of elements are compared and swapped if necessary. If the pair has the same value or is in ascending order, it is kept itself.
If there are N elements to sort, the bubble sort does N-1 to iterate through the array.
Let’s look at an example, how it works.
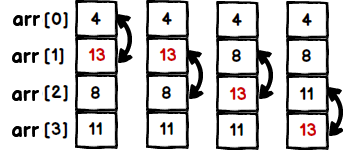
Bubble Sort in C
/* Bubble Sort in C */ #include <stdio.h> #define SIZE 10 int main() { int arr[10] = { 4, -1, 8, 12, -6, 23, 2, 28, 24, 33}; int i, j, tmp; //display the array elements for (i=0; i < SIZE; ++i) { printf("%4d", arr[i]); } for (i=0 ; i < SIZE-1; i++) { for (j=0 ; j < SIZE-i-1; j++) { /* For descending order use < operator */ if (arr[j] > arr[j+1]) { tmp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = tmp; } } } printf("\n******* Array Sorted in Ascending Order *******\n"); //display the elements of the sorted array for (i=0; i < SIZE; ++i) { printf("%4d", arr[i]); } return 0; }
Output:
4 -1 8 12 -6 23 2 28 24 33 ******* Array Sorted in Ascending Order ******* -6 -1 2 4 8 12 23 24 28 33