How to Get the First and Last Digit of a Number in C
John Howard
0 Comments
get the first and last digit of a number in c language, how to get first digit of a number in c, how to get last digit of a number in c, how to get the first digit of a number in c, how to print last digit of a number in c, write a c program to find first and last digit of a number
In this tutorial, we are going to see how to get the first and last digit of a number in C. The following C program, ask the user to enter a number and then find the first and last digits of the number without using loops in C programming language.
You should know how to use POW() function in C.
How to Get the First and Last Digit of a Number in C
#include <stdio.h> #include <math.h> int main() { int nbr, first, last, l; printf("\nEnter a number: "); scanf("%d", & nbr); // This will return the total number of digits - 1 l = log10(nbr); first = nbr / pow(10, l); last = nbr % 10; printf("\nThe first digit is %d", first); printf("\nThe last digit is %d", last); return 0; }
Output:
Enter a number: 123 The first digit is 1 The last digit is 3
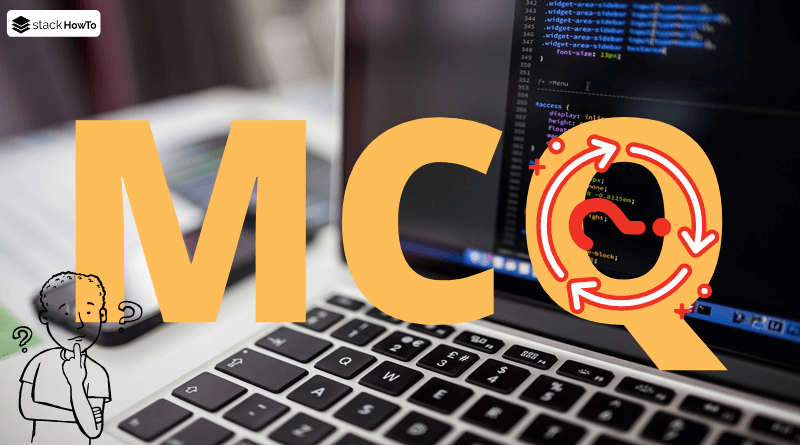
- 100 Multiple Choice Questions In C Programming – Part 1
- 100 Multiple Choice Questions In C Programming – Part 2
- 100 Multiple Choice Questions In C Programming – Part 3
- 100 Multiple Choice Questions In C Programming – Part 4
- 100 Multiple Choice Questions In C Programming – Part 5
- 100 Multiple Choice Questions In C Programming – Part 6
- 100 Multiple Choice Questions In C Programming – Part 7
- 100 Multiple Choice Questions In C Programming – Part 8
- 100 Multiple Choice Questions In C Programming – Part 9
- 100 Multiple Choice Questions In C Programming – Part 10
- 100 Multiple Choice Questions In C Programming – Part 11
- 100 Multiple Choice Questions In C Programming – Part 12
- 100 Multiple Choice Questions In C Programming – Part 13
- 100 Multiple Choice Questions In C Programming – Part 14
- Caesar Cipher Program in C
- Write a Program To Count Number of Words in String in C
- Perfect Number in C
- LCM of Two Numbers in C
- GCD Program in C Using Recursion
- GCD Program in C
- How to Get the First and Last Digit of a Number in C
- How to Use Pow in C Language
- C Program To Split a String Into Words
- C Program To Find Average Of N Numbers Using For Loop
- How to Print Double in C
- Multiplication Table Program in C Using For Loop
- C Program To Merge Two Files Into a Single File
- How To Delete a File in C
- C Program to Copy the Contents of One File to Another
- C Program To List All Files in a Directory
- How to Read a File in C
- How To Print Client IP Address in C
- C program to Display Current Date and Time
- C Program To Divide Two Complex Numbers Using Structures
- C Program To Multiply Two Complex Numbers Using Structures
- C Program To Subtract Two Complex Numbers Using Structures
- Write a C Program To Add Two Complex Numbers Using Structures
- C Program To Replace Multiple Spaces With Single Space
- Convert a String to Lowercase in C
- Convert a String to Uppercase in C Without toupper
- How to Extract a Substring From a String in C
- Matrix Multiplication in C
- Transpose of a Matrix in C
- Addition of Two Matrix in C
- Stack Implementation in C
- Sum of All Array Elements in C
- How To Print a 2D Array in C
- C Program To Remove Duplicate Elements in an Array
- C Program To Search an Element in an Array
- How To Merge Two Arrays in C
- How To Delete an Element From an Array in C
- How To Compare Two Strings in C Using For Loop Without strcmp
- How To Compare Two Strings in C Using strcmp
- How To Copy a String in C Using strcpy
- How To Copy a String in C Without Using strcpy
- How To Insert An Element At a Specific Position in Array
- How To Define, Declare, and Initialize a String in C
- How To Read String With Spaces in C
- Scanf String in C
- How To Find Length Of String In C
- How To Concatenate Two Strings In C Using Pointers
- How To Concatenate Two Strings In C Using Strcat
- How To Concatenate Two Strings In C Without Using Strcat
- Reverse a String In C Using Strrev Function
- Reverse a String In C Using Pointers
- Reverse a String In C Using Recursion
- Reverse a String In C Using For Loop
- Write a C Program To Reverse an Array Using Recursion
- Write a C Program To Reverse an Array Using Pointers
- Write a C Program To Reverse an Array Using Function
- Write a C Program To Reverse an Array Using For Loop
- How To Count The Number Of Occurrences Of a Character in a String in C
- How To Check If Two Strings Are Anagrams In C
- How To Generate Random Numbers in C With a Range
- C Program To Find Largest Number In An Array
- C Program To Find Smallest Number In An Array
- Inverted Pascal Triangle In C
- Pascal Triangle in C Using Array
- Pascal Triangle in C Using Function
- Pascal Triangle in C Using Recursion
- Pascal Triangle in C Using Factorial
- Pascal Triangle in C Using For Loop
- Armstrong Number in C
- How To Check Prime Number In C
- Merge Sort in C
- Quick Sort in C
- C Program To Print Square Star Pattern
- C Program To Print Inverted Triangle Star Pattern
- C Program To Convert Decimal To Binary Using For Loop
- C Program To Print Equilateral Triangle | Pyramid Star Pattern
- C Program To Find Largest and Smallest of Three Numbers Using Ternary Operator
- C Program To Find Largest Of 5 Numbers Using Ternary Operator
- C Program To Find Smallest Of N Numbers Using While Loop
- C Program To Find Largest Of N Numbers Using While Loop
- C Program To Find Largest Of 5 Numbers Using if-else
- C Program To Find Smallest Of 5 Numbers Using if-else
- Print 1 To 10 Using Recursion in C
- C Program To Print Even and Odd Numbers From 1 To 100
- C Program To Print Odd Numbers in a Given Range Using For Loop
- C Program To Print Even Numbers in a Given Range Using For Loop
- Write a Program to Check Even or Odd Numbers in C Using Function
- C Program to Print Even and Odd Numbers From 1 to N Using While Loop
- Write a Program to Print Even and Odd Numbers in C Using For Loop
- Reverse a Number in C using Recursive Function
- Reverse a Number in C using Function
- Reverse a Number in C using For Loop
- Reverse a Number in C using While Loop
- Leap Year Program in C
- Swapping Of Two Numbers In C Using Functions
- Swapping Of Two Numbers Without Temporary Variable in C
- Swap Two Numbers Using Temporary Variable in C
- Palindrome in C
- C Program to Check Whether an Alphabet is Vowel or Consonant Using Switch Case
- C Program to Check Whether an Alphabet is Vowel or Consonant Using Function
- C Program to Check Whether an Alphabet is Vowel or Consonant Using if-else
- C Program To Find Factorial Of a Number Using While Loop
- C Program To Find Factorial Of a Number Using For Loop
- C Program To Find Factorial Of a Number Using Function
- C Program To Find Factorial Of a Number Using Recursion
- Fibonacci Series In C Using Recursion
- Fibonacci Series In C Using For Loop
- Write a Program to Check Even or Odd Numbers in C Using if-else
- Write a Program to Add, Subtract, Multiply, and Divide Two Numbers in C
- C Program to Find Sum of Two Numbers
- Selection Sort in C
- Insertion Sort in C
- Bubble Sort in C