Pascal Triangle in C Using Recursion
In this tutorial, we are going to see how to display pascal triangle in C using recursion. Pascal’s triangle can be constructed by first placing a 1 along the left and right edges. Then the triangle can be filled from the top by adding the two numbers just above to the left and right of each position in the triangle.
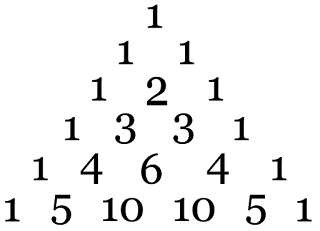
There are five ways to print pascal triangle in C, by using for loop, array, factorial, recursion, or by creating a function.
Pascal Triangle in C Using Recursion
#include <stdio.h> long pascalTriangle(int n, int i) { if(n == i || i == 0) return 1; else return pascalTriangle(n-1, i) + pascalTriangle(n-1, i-1); } int main() { int n, i, j, space = 0; printf("Enter the number of lines: "); scanf("%d", &n); for(i = 0; n >= i; i++) { for(space = 0; space < n-i; space++) printf(" "); for(j = 0; i >= j; j++) { long res = pascalTriangle(i, j); printf("%ld ", res); } printf("\n"); } return 0; }
Output:
Enter the number of lines: 7 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1 1 5 10 10 5 1 1 6 15 20 15 6 1