How to Make a Timer in Python Tkinter
In this tutorial, we are going to see how to make a timer in Python Tkinter. Tkinter provides a variety of built-in functions to create an interactive and functional GUI. The function after() is also a common function that can be used directly with the root window as well as with other widgets.
after(parent, ms, function = None, *args)
- parent: is the object of the widget or the main window, depending on who uses this function.
- ms: is the time in milliseconds.
- function: function that will be called.
- *args: other options.
How to Make a Timer in Python Tkinter
import tkinter as tk import time class Timer(): def __init__(self): self.root = tk.Tk() self.label = tk.Label(text="", font=('Times New Roman', 40)) self.label.pack() self.updateClock() self.root.mainloop() def updateClock(self): now = time.strftime("%H:%M:%S") self.label.configure(text = now) self.root.after(1000, self.updateClock) gui = Timer()
Output:
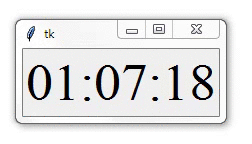