How to Get Window Size in Tkinter Python
In this tutorial, we are going to see how to get window size with Tkinter in Python. You can use the widget.winfo_width() function to get the width and widget.winfo_height() to get the height, but first you should call the widget’s update() function to find out the dimension. If you don’t call the update() function, you will get the default value 1.
How to Get Window Size in Tkinter Python
import tkinter as tk root = tk.Tk() root.geometry('250x300') root.update() # Get the width width = root.winfo_width() # Get the height height = root.winfo_height() print("Width: ", width) # Display the width print("Height: ", height) # Display the height root.mainloop()
Output:
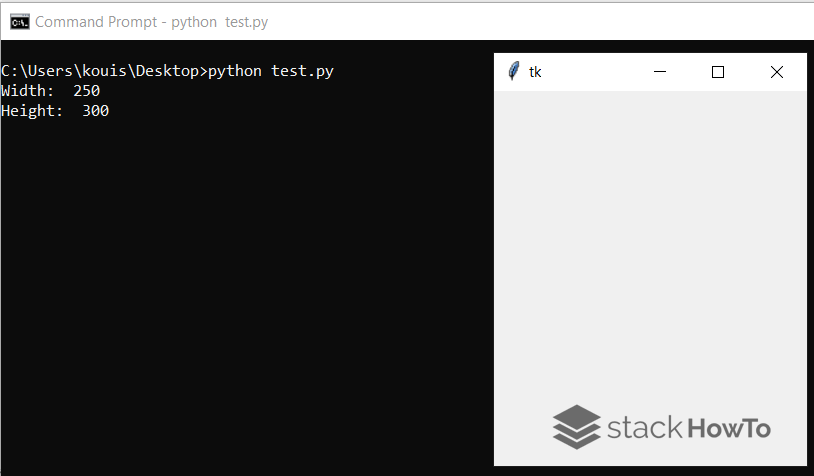