How to Disable/Enable Tkinter Button in Python
In this tutorial, we are going to see how to disable/enable Tkinter button in Python.
A Tkinter button has three states: active, normal, disabled.
You set the state option to “disabled” to grey out the button and make it insensitive. The button has the value “active” when the mouse is on it and the default value is “normal”.
Example 1: How to Disable Tkinter Button
from tkinter import * root = Tk() root.geometry('200x100') #Button 1: not clickable btn1 = Button(root, text = "Button 1", state = DISABLED) btn1.pack(side = LEFT) #Button 2: clickable btn2 = Button(root, text = "Button 2") btn2.pack(side = RIGHT) root.mainloop()
Output:
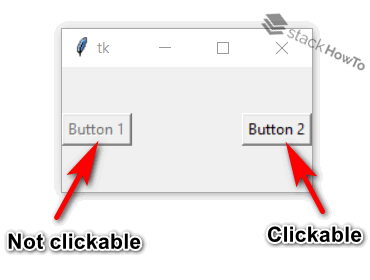
Example 2: How to Disable/Enable Tkinter Button
The states can be changed in the configuration method.
from tkinter import * def changeState(): if (btn1['state'] == NORMAL): btn1['state'] = DISABLED else: btn1['state'] = NORMAL root = Tk() root.geometry('300x100') #Button 1: not clickable btn1 = Button(root, text = "Button 1", state = DISABLED) btn1.pack(side = LEFT) #Bouton 2 : clickable btn2 = Button(root, text="Enable/Disable Btn 1", command=changeState) btn2.pack(side = RIGHT) root.mainloop()
Output:
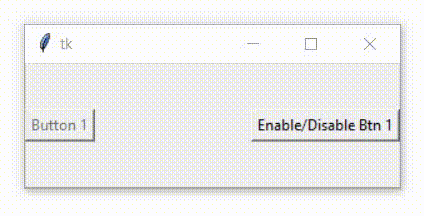